master
parent
c1737fc0a8
commit
5ddea02652
Binary file not shown.
Binary file not shown.
Binary file not shown.
@ -1,20 +0,0 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014 Brett Hagman
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
@ -1,137 +0,0 @@
|
||||
# SoftPWM Library #
|
||||
|
||||
----
|
||||
|
||||
## What's New? ##
|
||||
|
||||
Version 1.0.1
|
||||
|
||||
* Changes from Paul Stoffregen
|
||||
* Use IntervalTimer on Teensy 3.x
|
||||
* Use LED_BUILTIN for WLED in example
|
||||
|
||||
Version 1.0.0
|
||||
|
||||
* Initial release
|
||||
|
||||
## Description ##
|
||||
|
||||
A Wiring Framework (and Arduino) Library to produce PWM signals on any arbitrary pin.
|
||||
|
||||
It was originally designed for use controlling the brightness of LEDs, but could be modified to control servos and other low frequency PWM controlled devices as well.
|
||||
|
||||
It uses a single hardware timer (Timer 2 on AVR, or IntervalTimer on Teensy 3.x) on the microcontroller to generate up to 20 PWM channels.
|
||||
|
||||
----
|
||||
|
||||
## Features ##
|
||||
|
||||
* Arbitrary output pins
|
||||
* Up to 20 different channels can be created
|
||||
* True zero level, i.e. off == off
|
||||
* Separate fade rates for on and off
|
||||
|
||||
----
|
||||
|
||||
## Download and Installation ##
|
||||
|
||||
You can use the Arduino Library Manager (Sketch -> Include Library -> Manage Libraries...) to download the library.
|
||||
|
||||
Alternatively, you can download the library directly, and install it yourself.
|
||||
|
||||
* [SoftPWM Library - Latest Version](https://github.com/bhagman/SoftPWM/archive/master.zip)
|
||||
|
||||
Unzip the folder and rename it to `SoftPWM`, then move it to your `arduinosketchfolder/libraries/` folder.
|
||||
|
||||
----
|
||||
|
||||
## Usage Example ##
|
||||
|
||||
```
|
||||
#include "SoftPWM.h"
|
||||
|
||||
void setup()
|
||||
{
|
||||
// Initialize
|
||||
SoftPWMBegin();
|
||||
|
||||
// Create and set pin 13 to 0 (off)
|
||||
SoftPWMSet(13, 0);
|
||||
|
||||
// Set fade time for pin 13 to 100 ms fade-up time, and 500 ms fade-down time
|
||||
SoftPWMSetFadeTime(13, 100, 500);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
// Turn on - set to 100%
|
||||
SoftPWMSetPercent(13, 100);
|
||||
|
||||
// Wait for LED to turn on - you could do other tasks here
|
||||
delay(100);
|
||||
|
||||
// Turn off - set to 0%
|
||||
SoftPWMSetPercent(13, 0);
|
||||
|
||||
// Wait for LED to turn off
|
||||
delay(500);
|
||||
}
|
||||
```
|
||||
|
||||
----
|
||||
## Function Descriptions ##
|
||||
|
||||
`SoftPWMBegin([defaultPolarity])`
|
||||
|
||||
* Initializes the library - sets up the timer and other tasks.
|
||||
* optional `defaultPolarity` allows all newly defined pins to take on this polarity.
|
||||
* Values: `SOFTPWM_NORMAL`, `SOFTPWM_INVERTED`
|
||||
|
||||
`SoftPWMSet(pin,value)`
|
||||
|
||||
* `pin` is the output pin.
|
||||
* `value` is a value between 0 and 255 (inclusive).
|
||||
|
||||
`SoftPWMSetPercent(pin,percent)`
|
||||
|
||||
* `pin` is the output pin.
|
||||
* `percent` is a value between 0 and 100 (inclusive).
|
||||
|
||||
`SoftPWMSetFadeTime(pin,fadeUpTime,fadeDownTime)`
|
||||
|
||||
* `pin` is the output pin.
|
||||
* `fadeuptime` is the time in milliseconds that it will take the channel to fade from 0 to 255.
|
||||
* Range: 0 to 4000
|
||||
* `fadedowntime` is the time in milliseconds that it will take the channel to fade from 255 to 0.
|
||||
* Range: 0 to 4000
|
||||
|
||||
`SoftPWMSetPolarity(pin,polarity)`
|
||||
|
||||
* `pin` is the output pin.
|
||||
* `polarity` is the polarity for the given pin.
|
||||
|
||||
### Notes ###
|
||||
|
||||
* You can use `ALL` in place of the pin number to have the function act on all currently set channels.
|
||||
* e.g. `SoftPWMSetFadeTime(ALL, 100, 400)` - this will set all created channels to have a fade-up time of 100 ms and a fade-down time of 400.
|
||||
* The polarity setting of the pin is as follows:
|
||||
* `SOFTPWM_NORMAL` means that the pin is LOW when the PWM value is 0, whereas `SOFTPWM_INVERTED` indicates the pin should be HIGH when the PWM value is 0.
|
||||
|
||||
|
||||
----
|
||||
|
||||
## Demonstrations ##
|
||||
|
||||
Arduino Duemilanove LED Blink example - available as library example:
|
||||
|
||||
[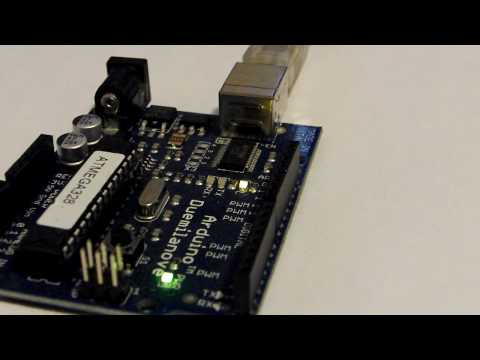](https://www.youtube.com/watch?v=9tTd7aLm9aQ)
|
||||
|
||||
rDuino LEDHead Bounce example - available as library example:
|
||||
|
||||
[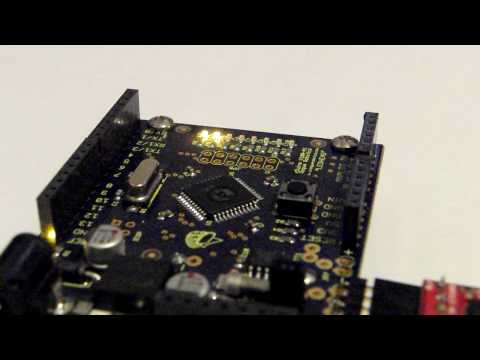](https://www.youtube.com/watch?v=jE7Zw1zNL6c)
|
||||
|
||||
More demos:
|
||||
|
||||
https://www.youtube.com/view_play_list?p=33BB5D2E20609C52
|
||||
|
||||
----
|
@ -1,13 +0,0 @@
|
||||
SoftPWM Library
|
||||
|
||||
Version Modified By Date Comments
|
||||
------- ------------ ---------- --------
|
||||
0001 B Hagman 2010-03-14 Initial coding (Pi Day!)
|
||||
0002 B Hagman 2010-03-21 License updates, minor fixes
|
||||
0003 B Hagman 2010-04-21 Added hardset control for syncing to matrix scan lines
|
||||
0004 B Hagman 2010-06-27 Fixed: pin 0 could not be used.
|
||||
0005 B Hagman 2011-05-27 Added polarity, and full Wiring support.
|
||||
B Hagman 2012-02-13 Fixed Arduino 1.0+ mess.
|
||||
1.0.0 B Hagman 2017-01-13 Initial release conforming to library standard.
|
||||
1.0.1 P Stoffregen 2017-08-30 Use IntervalTimer on Teensy 3.x and use LED_BUILTIN for WLED
|
||||
|
@ -1,18 +0,0 @@
|
||||
#include <SoftPWM.h>
|
||||
|
||||
void setup()
|
||||
{
|
||||
SoftPWMBegin();
|
||||
|
||||
SoftPWMSet(13, 0);
|
||||
|
||||
SoftPWMSetFadeTime(13, 1000, 1000);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
SoftPWMSet(13, 255);
|
||||
delay(1000);
|
||||
SoftPWMSet(13, 0);
|
||||
delay(1000);
|
||||
}
|
@ -1,38 +0,0 @@
|
||||
#include <SoftPWM.h>
|
||||
|
||||
#define DELAY 100
|
||||
uint8_t leds[8] = {22, 23, 26, 27, 28, 29, 30, 31};
|
||||
|
||||
void setup()
|
||||
{
|
||||
SoftPWMBegin();
|
||||
|
||||
for (int i = 0; i < 8; i++)
|
||||
SoftPWMSet(leds[i], 0);
|
||||
|
||||
SoftPWMSetFadeTime(ALL, 50, 400);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
int i;
|
||||
|
||||
for (i = 0; i < 7; i++)
|
||||
{
|
||||
SoftPWMSet(leds[i+1], 255);
|
||||
SoftPWMSet(leds[i], 0);
|
||||
delay(DELAY);
|
||||
}
|
||||
|
||||
delay(400);
|
||||
|
||||
for (i = 7; i > 0; i--)
|
||||
{
|
||||
SoftPWMSet(leds[i-1], 255);
|
||||
SoftPWMSet(leds[i], 0);
|
||||
delay(DELAY);
|
||||
}
|
||||
|
||||
delay(400);
|
||||
|
||||
}
|
@ -1,43 +0,0 @@
|
||||
#include <SoftPWM.h>
|
||||
|
||||
#define DELAY 40
|
||||
|
||||
uint8_t leds[8] = {22, 23, 26, 27, 28, 29, 30, 31};
|
||||
|
||||
void setup()
|
||||
{
|
||||
SoftPWMBegin();
|
||||
|
||||
for (int i = 0; i < 8; i++)
|
||||
SoftPWMSet(leds[i], 0);
|
||||
|
||||
SoftPWMSetFadeTime(ALL, 30, 200);
|
||||
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
int i;
|
||||
|
||||
for (i = 0; i < 3; i++)
|
||||
{
|
||||
SoftPWMSet(leds[i+1], 255);
|
||||
SoftPWMSet(leds[6-i], 255);
|
||||
SoftPWMSet(leds[i], 0);
|
||||
SoftPWMSet(leds[7-i], 0);
|
||||
delay(DELAY);
|
||||
}
|
||||
|
||||
delay(250);
|
||||
|
||||
for (i = 3; i > 0; i--)
|
||||
{
|
||||
SoftPWMSet(leds[i-1], 255);
|
||||
SoftPWMSet(leds[8-i], 255);
|
||||
SoftPWMSet(leds[i], 0);
|
||||
SoftPWMSet(leds[7-i], 0);
|
||||
delay(DELAY);
|
||||
}
|
||||
|
||||
delay(250);
|
||||
}
|
@ -1,24 +0,0 @@
|
||||
#include <SoftPWM.h>
|
||||
|
||||
#define DELAY 100
|
||||
uint8_t leds[8] = {22, 23, 26, 27, 28, 29, 30, 31};
|
||||
|
||||
void setup()
|
||||
{
|
||||
SoftPWMBegin();
|
||||
for (int i = 0; i < 8; i++)
|
||||
SoftPWMSet(leds[i], 0);
|
||||
|
||||
SoftPWMSetFadeTime(ALL, 50, 400);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
uint8_t pin = random(8);
|
||||
|
||||
SoftPWMSet(leds[pin], 255);
|
||||
delay(50);
|
||||
SoftPWMSet(leds[pin], 0);
|
||||
|
||||
delay(random(DELAY));
|
||||
}
|
@ -1,30 +0,0 @@
|
||||
#include <SoftPWM.h>
|
||||
|
||||
#ifndef WLED
|
||||
#define WLED LED_BUILTIN
|
||||
#endif
|
||||
|
||||
void setup()
|
||||
{
|
||||
SoftPWMBegin();
|
||||
|
||||
// Sets the PWM value to 0 for the built-in LED (WLED).
|
||||
SoftPWMSet(WLED, 0);
|
||||
|
||||
// Sets the default fade time for WLED to
|
||||
// 100 ms fade-up and 550 ms to fade-down.
|
||||
SoftPWMSetFadeTime(WLED, 100, 550);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
// Turn on WLED
|
||||
SoftPWMSet(WLED, 255);
|
||||
// Wait for the fade-up and some extra delay.
|
||||
delay(250);
|
||||
// Turn off WLED
|
||||
SoftPWMSet(WLED, 0);
|
||||
// Wait for the fade-down, and some extra delay.
|
||||
delay(650);
|
||||
}
|
||||
|
@ -1,36 +0,0 @@
|
||||
########################################
|
||||
# Syntax Coloring Map For SoftPWM
|
||||
########################################
|
||||
#
|
||||
# Fields (Data Members) - KEYWORD2
|
||||
# Methods (Member Functions)
|
||||
# - FUNCTION2 (or KEYWORD2)
|
||||
# Constants - LITERAL2
|
||||
# Datatypes - KEYWORD5 (or KEYWORD1)
|
||||
########################################
|
||||
|
||||
########################################
|
||||
# Datatypes (KEYWORD5 or KEYWORD1)
|
||||
########################################
|
||||
|
||||
SoftPWM KEYWORD1
|
||||
|
||||
########################################
|
||||
# Methods and Functions
|
||||
# (FUNCTION2 or KEYWORD2)
|
||||
########################################
|
||||
|
||||
SoftPWMSet KEYWORD2
|
||||
SoftPWMSetPercent KEYWORD2
|
||||
SoftPWMSetFadeTime KEYWORD2
|
||||
SoftPWMBegin KEYWORD2
|
||||
SoftPWMEnd KEYWORD2
|
||||
SoftPWMSetPolarity KEYWORD2
|
||||
|
||||
########################################
|
||||
# Constants (LITERAL2)
|
||||
########################################
|
||||
|
||||
ALL LITERAL2
|
||||
SOFTPWM_NORMAL LITERAL2
|
||||
SOFTPWM_INVERTED LITERAL2
|
@ -1,9 +0,0 @@
|
||||
name=SoftPWM
|
||||
version=1.0.1
|
||||
author=Brett Hagman <bhagman@wiring.org.co>
|
||||
maintainer=Brett Hagman <bhagman@wiring.org.co>
|
||||
sentence=A software library to produce a 50 percent duty cycle PWM signal on arbitrary pins.<br />
|
||||
paragraph=A Wiring Framework (and Arduino) Library, for Atmel AVR8 bit series microcontrollers and Teensy 3.x, to produce PWM signals on any arbitrary pin.<br />It was originally designed for controlling the brightness of LEDs, but could be adapted to control servos and other low frequency PWM controlled devices as well.<br />It uses a single hardware timer (Timer 2) on an Atmel AVR 8 bit microcontroller (or IntervalTimer on Teensy 3.x) to generate up to 20 PWM channels (your mileage may vary).<br /><br />Issues or questions: <a href="https://github.com/bhagman/SoftPWM/issues">https://github.com/bhagman/SoftPWM/issues</a><br />
|
||||
category=Signal Input/Output
|
||||
url=https://github.com/bhagman/SoftPWM
|
||||
architectures=avr,arm
|
@ -0,0 +1,102 @@
|
||||
#include <util/delay.h>
|
||||
#include <avr/io.h>
|
||||
#include <stdio.h>
|
||||
#include <stdarg.h>
|
||||
#include <util/setbaud.h>
|
||||
#include <Arduino.h>
|
||||
#include <lib.h>
|
||||
#include "SoftPWM.h"
|
||||
|
||||
#include <SPI.h>
|
||||
#include <nRF24L01.h>
|
||||
#include <RF24.h>
|
||||
|
||||
#define CE_PIN 3
|
||||
#define CS_PIN 2
|
||||
#define RED 4
|
||||
#define GREEN 5
|
||||
|
||||
class Radio {
|
||||
|
||||
public:
|
||||
RF24 instance;
|
||||
|
||||
};
|
||||
|
||||
class Motor {
|
||||
|
||||
public:
|
||||
int power;
|
||||
};
|
||||
|
||||
|
||||
const uint64_t dozer_pipe = 0xF0F0F0F0D2LL;
|
||||
const uint64_t remote_pipe = 0xF0F0F0F0E1LL;
|
||||
|
||||
struct StickValues {
|
||||
uint32_t stick_1_a;
|
||||
uint32_t stick_1_b;
|
||||
uint32_t stick_2_a;
|
||||
uint32_t stick_2_b;
|
||||
};
|
||||
|
||||
int main() {
|
||||
|
||||
pinMode(4, OUTPUT);
|
||||
pinMode(5, OUTPUT);
|
||||
|
||||
uart_init();
|
||||
SoftPWMBegin();
|
||||
|
||||
RF24 radio(CE_PIN, CS_PIN);
|
||||
radio.begin();
|
||||
radio.openWritingPipe(dozer_pipe);
|
||||
radio.openReadingPipe(1, remote_pipe);
|
||||
radio.startListening();
|
||||
|
||||
//digitalWrite(18, HIGH);
|
||||
// SoftPWMSet(18, 255, 1);
|
||||
|
||||
|
||||
digitalWrite(GREEN, HIGH);
|
||||
_delay_ms(2000);
|
||||
digitalWrite(GREEN, LOW);
|
||||
|
||||
bool breaking = false;
|
||||
int cycles = 0;
|
||||
while (!breaking) {
|
||||
|
||||
//unsigned long start_wait = micros();
|
||||
bool timeout = false;
|
||||
|
||||
while (!radio.available()) {
|
||||
if (cycles++ > 10) {
|
||||
digitalWrite(RED, HIGH);
|
||||
_delay_ms(50);
|
||||
digitalWrite(RED, LOW);
|
||||
_delay_ms(50);
|
||||
timeout = true;
|
||||
cycles = 0;
|
||||
break;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
if (timeout) {
|
||||
// SoftPWMSet(17, 0);
|
||||
// SoftPWMSet(18, 0);
|
||||
} else {
|
||||
|
||||
StickValues stick_values;
|
||||
radio.read(&stick_values, sizeof(uint32_t)*4);
|
||||
radio.flush_rx();
|
||||
radio.flush_tx();
|
||||
|
||||
// SoftPWMSet(17, stick_values.stick_1_a);
|
||||
// SoftPWMSet(18, stick_values.stick_1_b);
|
||||
}
|
||||
|
||||
SoftPWMSet(17, 80);
|
||||
SoftPWMSet(18, 120);
|
||||
}
|
||||
}
|
@ -1,169 +0,0 @@
|
||||
#include <util/delay.h>
|
||||
#include <avr/io.h>
|
||||
#include <stdio.h>
|
||||
#include <stdarg.h>
|
||||
#include <util/setbaud.h>
|
||||
#include <Arduino.h>
|
||||
#include <lib.h>
|
||||
#include "SoftPWM.h"
|
||||
|
||||
#include <SPI.h>
|
||||
#include <nRF24L01.h>
|
||||
#include <RF24.h>
|
||||
|
||||
#define CE_PIN 3
|
||||
#define CS_PIN 2
|
||||
#define RED 4
|
||||
#define GREEN 5
|
||||
|
||||
class Radio {
|
||||
|
||||
public:
|
||||
RF24 instance;
|
||||
|
||||
};
|
||||
|
||||
class Motor {
|
||||
|
||||
public:
|
||||
int power;
|
||||
};
|
||||
|
||||
const uint64_t pipes[2] = {0xF0F0F0F0E1LL, 0xF0F0F0F0D2LL};
|
||||
|
||||
int radio_number = ROLE;
|
||||
int role = ROLE;
|
||||
|
||||
int main(void) {
|
||||
|
||||
pinMode(4, OUTPUT);
|
||||
pinMode(5, OUTPUT);
|
||||
|
||||
uart_init();
|
||||
SoftPWMBegin();
|
||||
|
||||
// SoftPWMSetFadeTime(4, 2000, 2000);
|
||||
// pinMode(15, OUTPUT);
|
||||
//
|
||||
// pinMode(16, OUTPUT);
|
||||
// pinMode(17, OUTPUT);
|
||||
//
|
||||
// pinMode(18, OUTPUT);
|
||||
// pinMode(19, OUTPUT);
|
||||
|
||||
|
||||
RF24 radio(CE_PIN, CS_PIN);
|
||||
radio.begin();
|
||||
|
||||
if (radio_number) {
|
||||
radio.openWritingPipe(pipes[1]);
|
||||
radio.openReadingPipe(1, pipes[0]);
|
||||
} else {
|
||||
radio.openWritingPipe(pipes[0]);
|
||||
radio.openReadingPipe(1, pipes[1]);
|
||||
}
|
||||
|
||||
radio.startListening();
|
||||
|
||||
|
||||
SoftPWMSet(17, 255);
|
||||
SoftPWMSet(18, 255);
|
||||
|
||||
|
||||
//digitalWrite(18, HIGH);
|
||||
// SoftPWMSet(18, 255, 1);
|
||||
// digitalWrite(19, LOW);
|
||||
|
||||
// digitalWrite(14, LOW);
|
||||
// digitalWrite(15, HIGH);
|
||||
|
||||
digitalWrite(GREEN, HIGH);
|
||||
_delay_ms(200);
|
||||
digitalWrite(GREEN, LOW);
|
||||
|
||||
int accum = 20;
|
||||
int i = 5;
|
||||
for (;;) {
|
||||
|
||||
unsigned long start_time = micros();
|
||||
|
||||
// ping
|
||||
if (role == 1) {
|
||||
|
||||
|
||||
if (accum > 230) {
|
||||
i = -5; accum = 230;
|
||||
} else if (accum < 100) {
|
||||
i = 5; accum = 100;
|
||||
}
|
||||
accum += i;
|
||||
|
||||
// SoftPWMSet(14, accum);
|
||||
// SoftPWMSet(19, accum);
|
||||
_delay_ms(100);
|
||||
//
|
||||
//// digitalWrite(GREEN, HIGH);
|
||||
//// _delay_ms(200);
|
||||
//// digitalWrite(GREEN, LOW);
|
||||
// radio.stopListening();
|
||||
//
|
||||
//
|
||||
// if (!radio.write(&start_time, sizeof(unsigned long))) {
|
||||
//// digitalWrite(RED, HIGH);
|
||||
//// _delay_ms(1000);
|
||||
//// digitalWrite(RED, LOW);
|
||||
// radio.startListening();
|
||||
// continue;
|
||||
// }
|
||||
//
|
||||
// radio.startListening();
|
||||
// unsigned long started_waiting_at = micros();
|
||||
// boolean timeout = false;
|
||||
//
|
||||
// _delay_ms(390);
|
||||
// if (!radio.available()) {
|
||||
// timeout = true;
|
||||
// }
|
||||
//// while (!radio.available()) {
|
||||
//// if (micros() - started_waiting_at > 20) {
|
||||
//// timeout = true;
|
||||
//// break;
|
||||
//// }
|
||||
//// }
|
||||
// if (timeout) {
|
||||
//
|
||||
//// digitalWrite(RED, HIGH);
|
||||
//// _delay_ms(200);
|
||||
//// digitalWrite(RED, LOW);
|
||||
//
|
||||
// } else {
|
||||
// unsigned long got_time;
|
||||
// radio.read(&got_time, sizeof(unsigned long));
|
||||
// unsigned long end_time = micros();
|
||||
//
|
||||
//// digitalWrite(GREEN, HIGH);
|
||||
//// _delay_ms(200);
|
||||
//// digitalWrite(GREEN, LOW);
|
||||
// }
|
||||
|
||||
|
||||
//_delay_ms(500);
|
||||
|
||||
}
|
||||
|
||||
// ping bag
|
||||
if (role == 0) {
|
||||
_delay_ms(100);
|
||||
unsigned long got_time;
|
||||
if (radio.available()) {
|
||||
while (radio.available()) {
|
||||
radio.read(&got_time, sizeof(unsigned long));
|
||||
}
|
||||
radio.stopListening();
|
||||
radio.write(&got_time, sizeof(unsigned long));
|
||||
radio.startListening();
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -0,0 +1,81 @@
|
||||
#include <util/delay.h>
|
||||
#include <avr/io.h>
|
||||
#include <stdio.h>
|
||||
#include <stdarg.h>
|
||||
#include <util/setbaud.h>
|
||||
#include <Arduino.h>
|
||||
#include <lib.h>
|
||||
#include "SoftPWM.h"
|
||||
|
||||
#include <SPI.h>
|
||||
#include <nRF24L01.h>
|
||||
#include <RF24.h>
|
||||
|
||||
#define CE_PIN 3
|
||||
#define CS_PIN 2
|
||||
#define RED 4
|
||||
#define GREEN 5
|
||||
|
||||
class Radio {
|
||||
|
||||
public:
|
||||
RF24 instance;
|
||||
|
||||
};
|
||||
|
||||
class Motor {
|
||||
|
||||
public:
|
||||
int power;
|
||||
};
|
||||
|
||||
const uint64_t dozer_pipe = 0xF0F0F0F0D2LL;
|
||||
const uint64_t remote_pipe = 0xF0F0F0F0E1LL;
|
||||
|
||||
struct StickValues {
|
||||
uint32_t stick_1_a;
|
||||
uint32_t stick_1_b;
|
||||
uint32_t stick_2_a;
|
||||
uint32_t stick_2_b;
|
||||
};
|
||||
|
||||
int main() {
|
||||
|
||||
pinMode(4, OUTPUT);
|
||||
pinMode(5, OUTPUT);
|
||||
|
||||
uart_init();
|
||||
|
||||
RF24 radio(CE_PIN, CS_PIN);
|
||||
radio.begin();
|
||||
radio.openWritingPipe(remote_pipe);
|
||||
radio.openReadingPipe(1, dozer_pipe);
|
||||
radio.startListening();
|
||||
|
||||
bool breaking = false;
|
||||
while (!breaking) {
|
||||
|
||||
_delay_ms(100);
|
||||
|
||||
// Get input
|
||||
|
||||
// Parse into a struct
|
||||
StickValues stick_values;
|
||||
stick_values.stick_1_a = 100;
|
||||
stick_values.stick_1_b = 50;
|
||||
stick_values.stick_2_a = 0;
|
||||
stick_values.stick_2_b = 0;
|
||||
|
||||
// Send to dozer
|
||||
radio.stopListening();
|
||||
radio.write(&stick_values, sizeof(uint32_t)*4);
|
||||
radio.startListening();
|
||||
}
|
||||
}
|
||||
|
||||
// Dot really ever have to read
|
||||
//if (radio.available()) {
|
||||
// while (radio.available()) {
|
||||
// radio.read(&got_time, sizeof(unsigned long));
|
||||
// }
|
||||
//}
|
Loading…
Reference in new issue